JSON parser
IoT Toolkit
The JSON parser library is part of the IoT toolkit that allows easy integration and handling of the JSON (JavaScript Object Notation) data interchange format in an application.
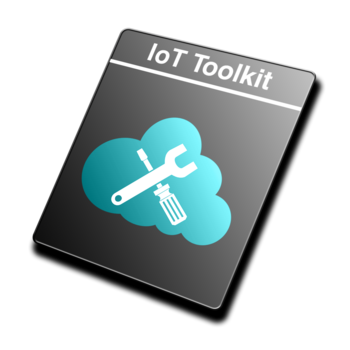
Overview
The JSON parser library is part of the IoT toolkit that allows easy integration and handling of the JSON (JavaScript Object Notation) data interchange format in an application without having to deal with the details of it. This allows you to easily evaluate the data returned by modern IoT REST APIs.
The JSON data interchange format is now widely agreed as the standard data format for modern IoT applications and their REST APIs. While other formats like XML provide a similar way to present data, the JSON format is more lightweight while still providing a (human readable) textual representation that is easy to process.
Why SEGGER's JSON Parser?
SEGGER's JSON parser provides a simple to use API that allows you to parse received JSON data without having to know the details of the format. As the JSON data interchange format is used by most modern REST APIs it is a must have component if you are working with IoT applications.
Parsing the tree like JSON data is achieved without actually building other tree like structures for internal processing. This not only benefits the overall RAM requirement but clever internal processing also avoids code recursion to make the stack space bounded.
Sample applications and demos are available to show how simple it is to parse JSON data and how the parsed data is delivered to your application for further processing.
SEGGER has developed the JSON parser from scratch to keep stack usage as small as possible and memory requirements to a minimum.
Key features
- Memory efficient and easy to use API
- Supports parsing JSON data in a space-efficient manner
- Incremental parsing of JSON data read in chunks
- Items are returned as events instead of a tree-like structure
- No recursive calls for nested JSON objects, so stack space is bounded
Easy to use API
Using the JSON parser API, handling a response received with JSON data is done in only a couple of lines of simple code.
static const char _sJSONValue[] = {
"{ "
" \"name\": \"My TV\", "
" \"resolutions\": [ "
" { \"width\": 1280, \"height\": 720 }, "
" { \"width\": 1920, \"height\": 1080 }, "
" { \"width\": 3840, \"height\": 2160 } "
" ] "
"} "
};
static const IOT_JSON_EVENTS _EventAPI = {
_BeginObject,
_EndObject,
_BeginArray,
_EndArray,
_Key,
_String,
_Number,
_Literal
};
void MainTask(void) {
IOT_JSON_CONTEXT JSON;
char acBuf[32];
//
IOT_JSON_Init(&JSON, &_EventAPI, &acBuf[0], sizeof(acBuf));
if (IOT_JSON_Parse(&JSON, _sJSONValue, sizeof(_sJSONValue)-1) == 0) {
printf("\nParse OK\n");
} else {
printf("\nParse FAIL\n");
}
}
Requirements
The JSON parser itself does not rely on any external components. The shipment includes source code samples and Win32 binaries of the samples showing different examples on how to handle data parsed by the API.
The library requires a small work buffer which size is actually determined by the size of the tokens found in the JSON object to parse. As most tokens only consist of a couple of characters even a small buffer like 32 bytes can already be sufficient.
The SEGGER IoT Toolkit evaluation package
A collection of free Windows executables is available for evaluation purposes to show the functionality of the components of the IoT Toolkit.
You can download the IoT Toolkit evaluation package from the following location: IoT Toolkit evaluation package
JSON parser samples
The following is an excerpt of the samples that can be downloaded with the IoT Toolkit evaluation package in binary form. The shipment also includes the source code to these samples.
Parsing JSON data using IOT_JSON_Print.exe
This executable shows processing of data that would be returned by an IoT REST resource. Today's standard for data retrieved and sent to REST APIs is the JSON data format. The sample allows you to test that your JSON data can actually be parsed by the IoT Toolkit JSON library and be utilized in your product.
The sample reads a JSON data content from the standard input of your console. The file "JSON_Content.txt" contains JSON example data as it would be returned by a REST API resource accessed with an HTTP client.
To feed the test data to the executable please execute the following command line:
IOT_JSON_Print.exe < JSON_Content.txt