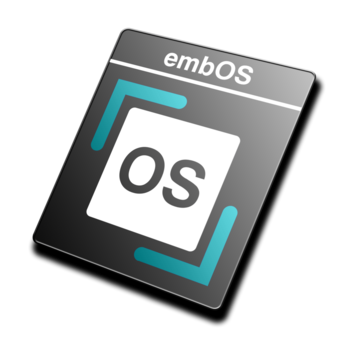
Overview
The embOS tickless low power support reduces power consumption, which is particularly important for battery powered devices. Instead of having a timer interrupt for each system tick, the timer is reprogrammed in order to spend as much time as possible in low power mode.
Tickless support can be added to any embOS start project. The embOS tickless support is based on three API functions: OS_TICKLESS_GetNumIdleTicks(), OS_TICKLESS_Start() and OS_TICKLESS_AdjustTime().
In order to use the tickless support, the OS_Idle() function has to be modified and a callback function has to be implemented. The OS_Idle() function calculates the amount of time which may be spent in low power mode and reprograms the hardware timer accordingly. The callback function is called automatically by the scheduler when the low power period expires. It adjusts the system time and resets the hardware timer to its default tick time.
Example implementation
void OS_Idle(void) {
OS_TIME IdleTicks;
OS_INT_Disable();
IdleTicks = OS_TICKLESS_GetNumIdleTicks();
if (IdleTicks > 1u) {
if ((OS_U32)IdleTicks > TIM2_MAX_TICKS) {
IdleTicks = TIM2_MAX_TICKS;
}
OS_TICKLESS_Start(IdleTicks, &_EndTicklessMode);
TIM2_ARR = (OS_TIMER_RELOAD * IdleTicks) - TIM2_CNT; // Set compare reg.
}
OS_INT_Enable();
while (1) {
__asm(" wfi");
}
}
static void _EndTicklessMode(void) {
OS_U16 NumTicks;
OS_U16 Cnt;
OS_U16 IReq;
if (OS_Global.TicklessExpired != 0) {
// The timer interrupt was executed => we completed the sleep time
OS_TICKLESS_AdjustTime(OS_Global.TicklessFactor);
} else {
Cnt = TIM2_CNT;
IReq = TIM2_SR & (1u << 0);
if (IReq) {
OS_TICKLESS_AdjustTime(OS_Global.TicklessFactor);
} else {
//
// We assume OS_TIMER_RELOAD counts per tick and hardware timer
// which counts up.
//
NumTicks = Cnt / OS_TIMER_RELOAD;
Cnt -= (NumTicks * OS_TIMER_RELOAD);
TIM2_CNT = Cnt;
OS_TICKLESS_AdjustTime(NumTicks);
}
}
TIM2_ARR = OS_TIMER_RELOAD; // Set default value for 1 tick
}
Example project
SEGGER offers Tickless demos for STM32L476 and nRF52832. Please visit SEGGER Wiki for detailed information on the example projects.