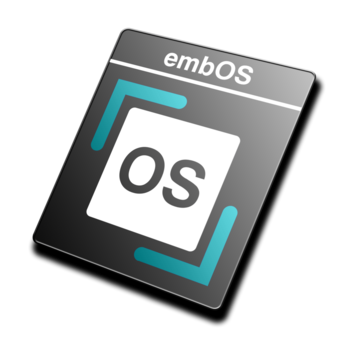
embOS supports cycle precise run-time measurements that may be used for calculating timings in your application.
embOS offers API functions to retrieve the current system time in timer cycle resolution.The actual resolution depends on your hardware timer frequency. For example if your hardware timer runs at 400 MHz, one timer cycle equals 1 sec / 400.000.000 cycles = 2,5 nano seconds per cycle.
This feature is easy to use and can be utilized with any embOS board support package. An application merely needs to set up this feature with a simple API call to OS_TIME_ConfigSysTimer(). This is already done for most embOS board support package, and may swiftly be done for any further embOS board support package on request.
OS_TIME_GetCycles() returns the current system time in timer cycles as a 64-bit value:
void MeasureTime(void) {
OS_U64 tStart
OS_U64 tEnd;
tStart = OS_TIME_GetCycles();
DoSomeThing();
tEnd= OS_TIME_GetCycles();
printf("Time to run the function: %u cycles", tEnd - tStart);
}
With this 64-bit data type OS_TIME_GetCycles() can return up to 0xFFFFFFFFFFFFFFFF cycles (~18 quintillion cycles). That ensures you can even measure very long time periods without any overflow. For example with the above timer frequency of 400 MHz you can measure time periods up to ~1462 years.
embOS offers API functions to convert the timer cycles into nano seconds or micro seconds:
void Convert(void) {
OS_U64 cycles;
OS_U64 nano_sec;
OS_U64 micro_sec;
cycles = OS_TIME_GetCycles();
nano_sec = OS_TIME_ConvertCycles2ns(cycles);
micro_sec = OS_TIME_ConvertCycles2us(cycles);
}
embOS also offers API functions for micro second precise timings:
void MeasureTime(void) {
OS_U64 tStart
OS_U64 tEnd;
tStart = OS_TIME_Getus64();
DoSomeThing();
tEnd = OS_TIME_Getus64();
printf("Time to run the function: %u micro seconds", tEnd - tStart);
}