WebUSB access
Access your emUSB-Device target via JavaScript from a web browser.
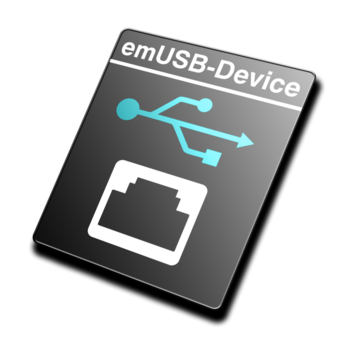
What is WebUSB
WebUSB is an API standard to provide access to USB devices from web pages. WebUSB is developed by Google.
WebUSB can be used by a web page to communicate with a USB device which is connected to the user's PC.
This works seamlessly with emUSB-Device.
Example
The example on the right demonstrates a web page with 4 buttons which allow the LEDs on an evaluation board to be toggled on and off. The example uses WebUSB and the emUSB-Device-Bulk component.
Download Demo for the emPower board.
To try WebUSB with emUSB-Device download the above package, program the emPower board with the included .srec file, connect the USB cable and click connect. After that you will be able to control the board's LEDs with the buttons on the right.
For the sake of clarity the sample is separated into two JavaScript files and one web page which includes both and contains the HTML code for the buttons. The first JavaScript file "seggerbulk.js" describes the emUSB-Device-Bulk target and is used by WebUSB to find the device among the other USB devices connected to the PC. The second file "led.js" is responsible for sending the buttons status to our target.
The most important part of the first JavaScript file is the detection of the target running emUSB-Device-Bulk. This is done by creating a filter for the vendor and product IDs:
function _Connect() { var PromiseObj; _USBDev = new SeggerBulk({'vendorId': 0x8765, 'productId': 0x1240}); PromiseObj = _USBDev.Connect(); PromiseObj.then(_cbOnConnect, _cbOnError); }
The JavaScript for the buttons contains a simple function which sends the LED status to the device on each click of a button:
function _cbOnLEDUpdate() { if (!_USBDev) { return; } var view = new Uint8Array(4); view[0] = _aLED[0].checked ? 1 : 0; view[1] = _aLED[1].checked ? 1 : 0; view[2] = _aLED[2].checked ? 1 : 0; view[3] = _aLED[3].checked ? 1 : 0; _USBDev.Send(view, null, _cbOnError); _USBDev.Receive(_cbOnDataReceived, _cbOnError); }
Try it now!
Additional information
- WebUSB API description https://wicg.github.io/webusb
- List of browsers with WebUSB support https://caniuse.com/#search=webusb